Export Flutter animations
Here's how to export mobile app animations with Flutter. Learn how to create Flutter animations following these instructions:
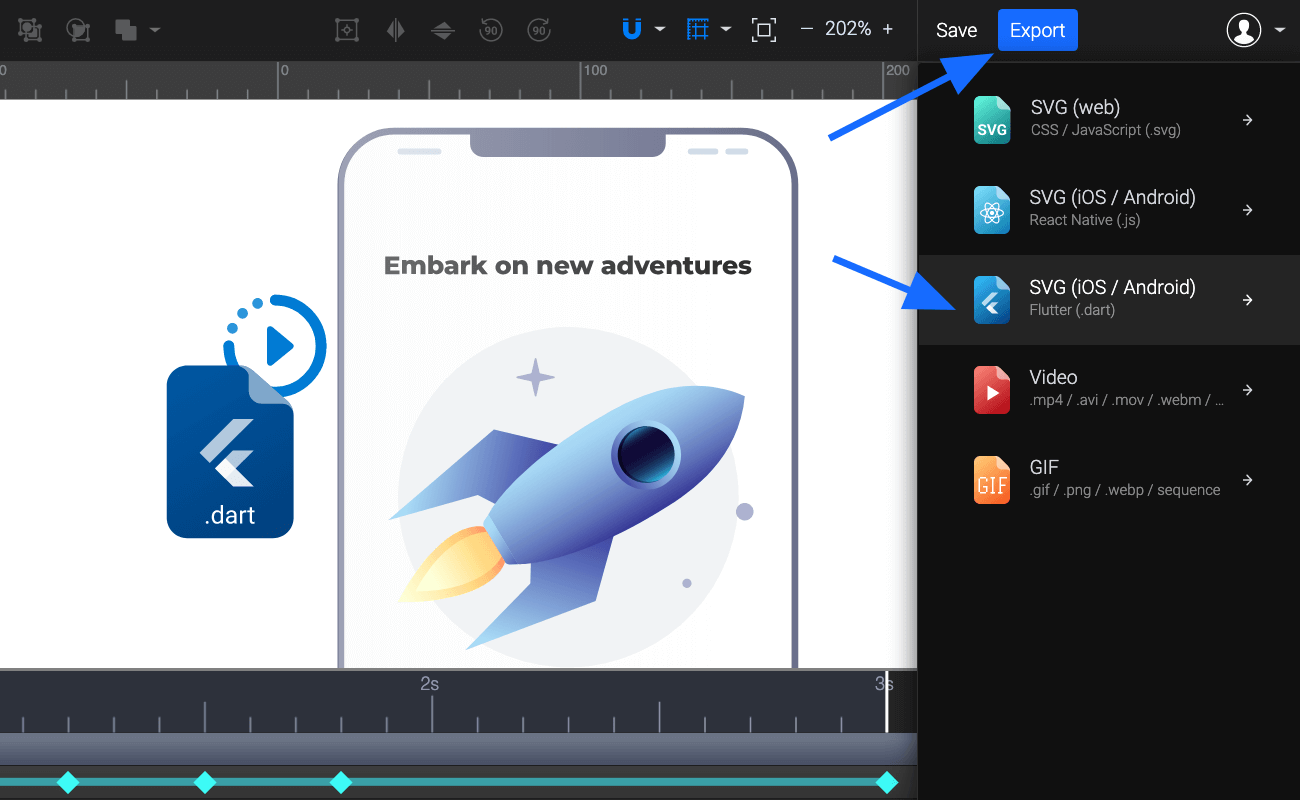
Requirements
- webview_flutter module (link)
- (optional - for single-player setup) SVGator's Flutter wrapper class
How to install webview_flutter
Inside your project’s root directory, run the following command:
flutter pub add webview_flutter
That’s all. You should be able to use our exported file.
How to use the exported file
- Copy the downloaded file into your project
- Include it into the component you are willing to use, as follows:
Original file:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
final _expandedKey = UniqueKey();
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: const Text('Welcome to Flutter'),
),
body: Column(
children: [
],
)
),
);
}
}
New file:
import 'package:flutter/material.dart';
import 'SVGator.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
final _expandedKey = UniqueKey();
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: const Text('Welcome to Flutter'),
),
body: Column(
children: [
SVGator()
],
)
),
);
}
}
IMPORTANT! The class name is derived from the file name used during the export, so if you want to use a different name, you will have to rename both the fine name and the class name.
Single-player setup
- The player JS will be loaded from a common location: SVGator's Flutter wrapper class
You will have to add our Flutter animation player as dependency to your project.
For this, edit your pubspec.yaml file by adding the dependency in red:
Version: 1.0.0+1
environment:
sdk: ">=2.12.0 <3.0.0"
dependencies:
flutter:
sdk: flutter
svgator_player_flutter: ^1.0.3 #add this to your pubspec.yaml file
webview_flutter: ^2.0.0
cupertino_icons: ^1.0.2
english_words: ^4.0.0
dev_dependencies:
flutter_test:
sdk: flutter
Note that the webview_flutter package will be still needed for the exported file.
Limitations
- Due to the way webview_flutter is implemented, our Flutter animations can't have transparent background.
Note that this is a known issue in the flutter community and everyone is waiting for a perfect solution inside the webview_flutter package.
More articles:
Export React Native animations
Still got questions? Send us an email at contact@svgator.com and we will get back to you as soon as we can.